433Mhz Test
Fleeting433Mhz test FS1000A and mx-rm-5v
using raspberry pi
trying https://www.instructables.com/Super-Simple-Raspberry-Pi-433MHz-Home-Automation/
I plugged the receiver on the GPIO 4 and the transmitter on the GPIO 17.
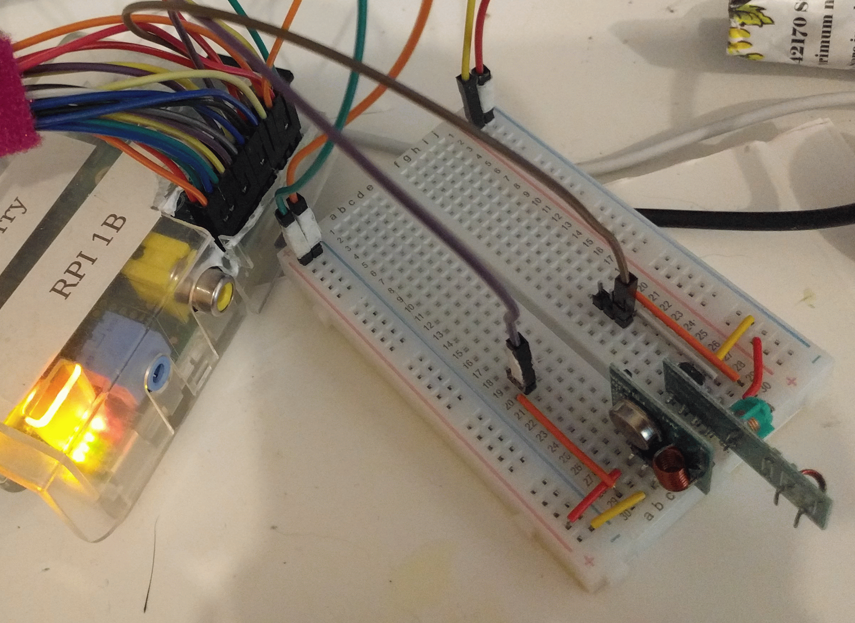
Using the given code, adjusted to my pins.
from datetime import datetime
import RPi.GPIO as GPIO
from collections import Counter
MAX_DURATION = 10
RECEIVE_PIN = 4
def capture():
global RECEIVED_SIGNAL
RECEIVED_SIGNAL = [[], []] # [[time of reading], [signal reading]]
GPIO.setmode(GPIO.BCM)
GPIO.setup(RECEIVE_PIN, GPIO.IN)
cumulative_time = 0
beginning_time = datetime.now()
print('**Started recording**')
while cumulative_time < MAX_DURATION:
time_delta = datetime.now() - beginning_time
RECEIVED_SIGNAL[0].append(time_delta)
RECEIVED_SIGNAL[1].append(GPIO.input(RECEIVE_PIN))
cumulative_time = time_delta.seconds
print('**Ended recording**')
print(len(RECEIVED_SIGNAL[0]), 'samples recorded')
GPIO.cleanup()
print('**Processing results**')
for i in range(len(RECEIVED_SIGNAL[0])):
RECEIVED_SIGNAL[0][i] = RECEIVED_SIGNAL[0][i].seconds + RECEIVED_SIGNAL[0][i].microseconds/1000000.0
print(f"Received: {Counter(RECEIVED_SIGNAL[1])}")
def dump(path="data/a.csv"):
Path(path).write_text("".join(f"{a},{b}\n" for a, b in zip(*RECEIVED_SIGNAL)))
def plot():
import matplotlib.pyplot as pyplot
print '**Plotting results**'
pyplot.plot(RECEIVED_SIGNAL[0], RECEIVED_SIGNAL[1])
pyplot.axis([0, MAX_DURATION, -1, 2])
pyplot.show()
def main():
capture()
plot()
if __name__ == "__main__":
main()
trying without doing anything with my devices
I already get a lot of data.
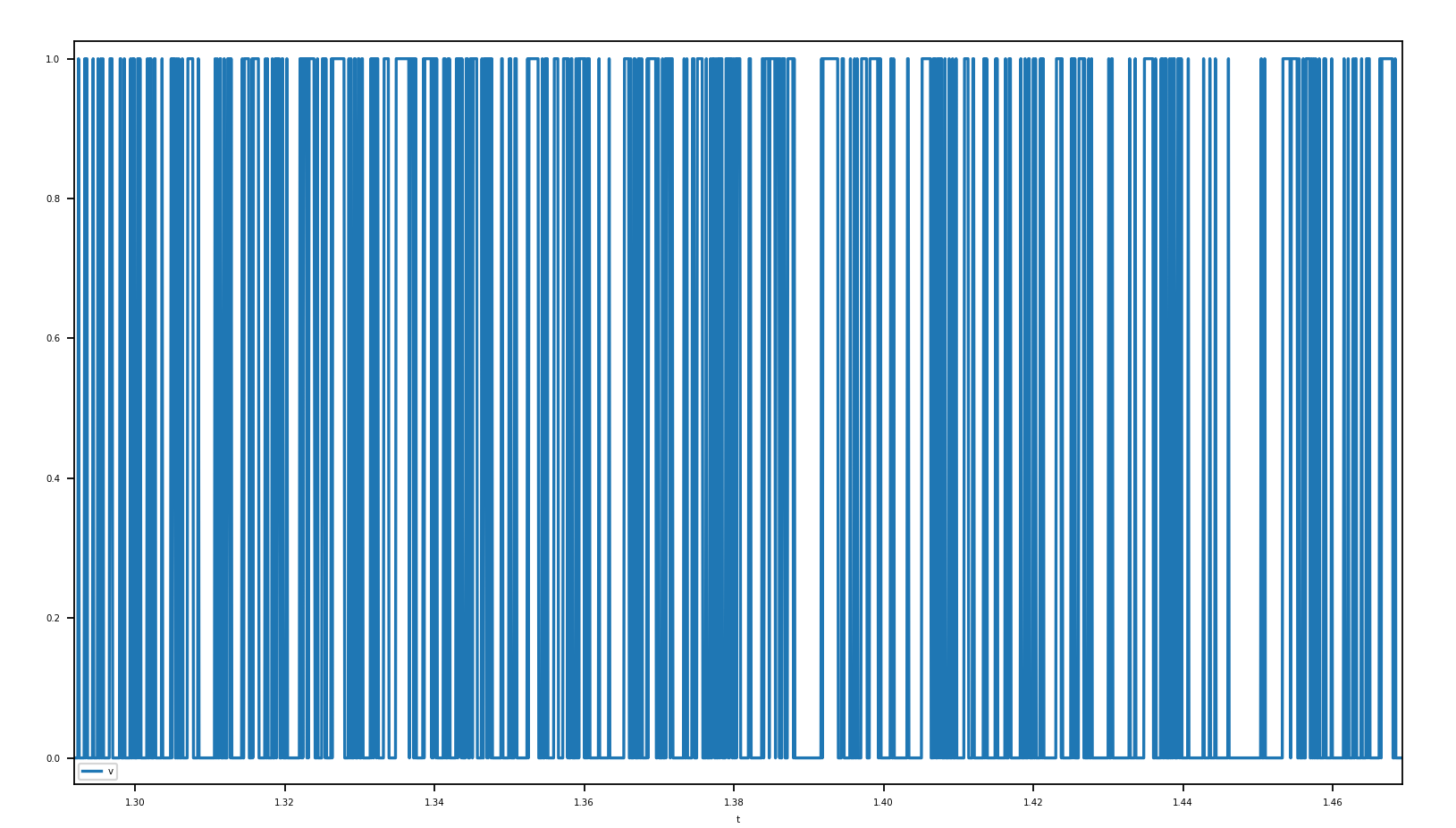
There are maybe some devices that send those data and that I don’t know about.
trying a base scenario without anything
I try unplugging the receiver.
trying with another library
https://www.instructables.com/RF-433-MHZ-Raspberry-Pi/
using arduino uno r3
Let’s try to follow the example shown in https://www.instructables.com/Arduino-433Mhz-Wireless-Communication-Rc-Switch/.
This is the wiring:
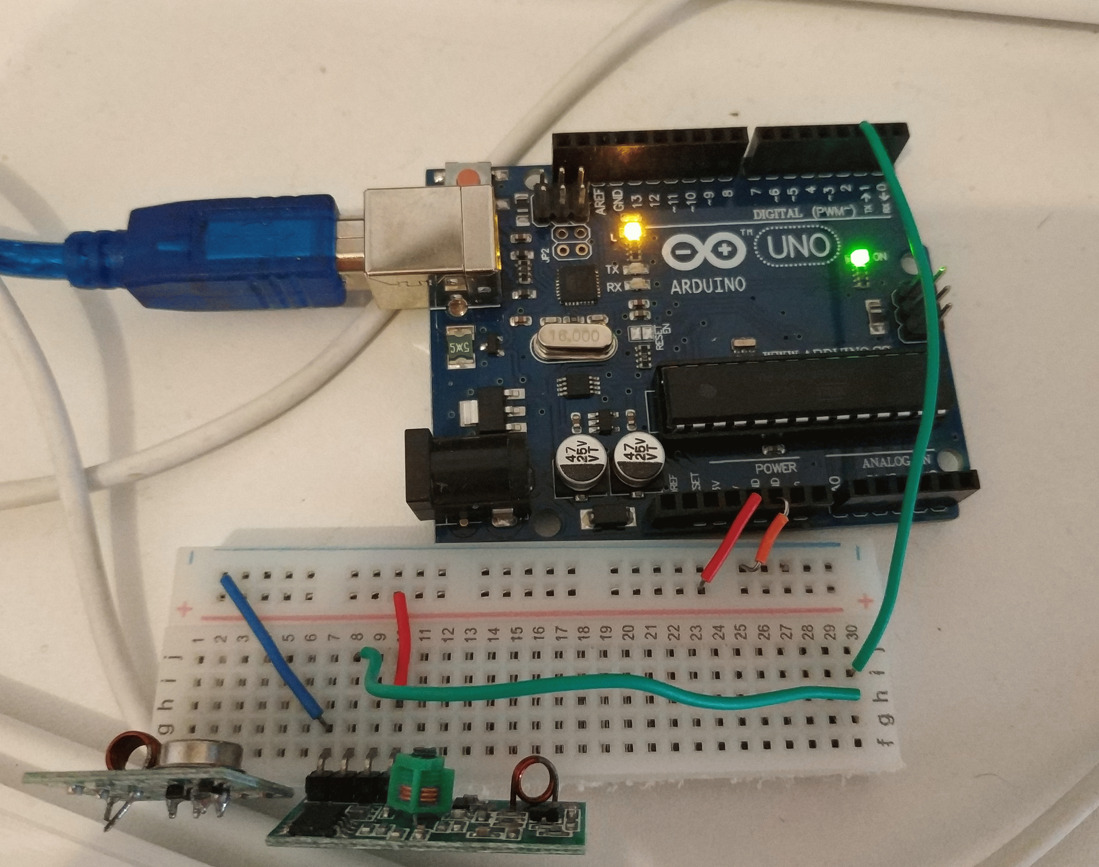
arduino-cli lib search rcswitch
arduino-cli lib install rc-switch
Downloading rc-switch@2.6.4...
rc-switch@2.6.4 0 B / 20.47 KiB 0.00%
rc-switch@2.6.4 downloaded
Installing rc-switch@2.6.4...
Installed rc-switch@2.6.4
See the examples in https://github.com/sui77/rc-switch/tree/master/examples
Here, let’s use the code from https://github.com/sui77/rc-switch/blob/master/examples/ReceiveDemo_Simple/ReceiveDemo_Simple.ino
#include <RCSwitch.h>
RCSwitch mySwitch = RCSwitch();
void setup() {
Serial.begin(9600);
Serial.print("Setting up");
mySwitch.enableReceive(0); // Receiver on interrupt 0 => that is pin #2
}
void loop() {
if (mySwitch.available()) {
Serial.print("Received ");
Serial.print( mySwitch.getReceivedValue() );
Serial.print(" / ");
Serial.print( mySwitch.getReceivedBitlength() );
Serial.print("bit ");
Serial.print("Protocol: ");
Serial.println( mySwitch.getReceivedProtocol() );
mySwitch.resetAvailable();
}
}
Sketch uses 3114 bytes (9%) of program storage space. Maximum is 32256 bytes.
Global variables use 401 bytes (19%) of dynamic memory, leaving 1647 bytes for local variables. Maximum is 2048 bytes.
[92mUsed library[0m [92mVersion[0m [90mPath[0m
[93mrc-switch[0m 2.6.4 [90m/home/sam/Arduino/libraries/rc-switch[0m
[92mUsed platform[0m [92mVersion[0m [90mPath[0m
[93marduino:avr[0m 1.8.6 [90m/home/sam/.arduino15/packages/arduino/hardware/avr/1.8.6[0m
New upload port: /dev/ttyACM1 (serial)
I have a DI-O chacon shutter remote that I can use to check my setup.
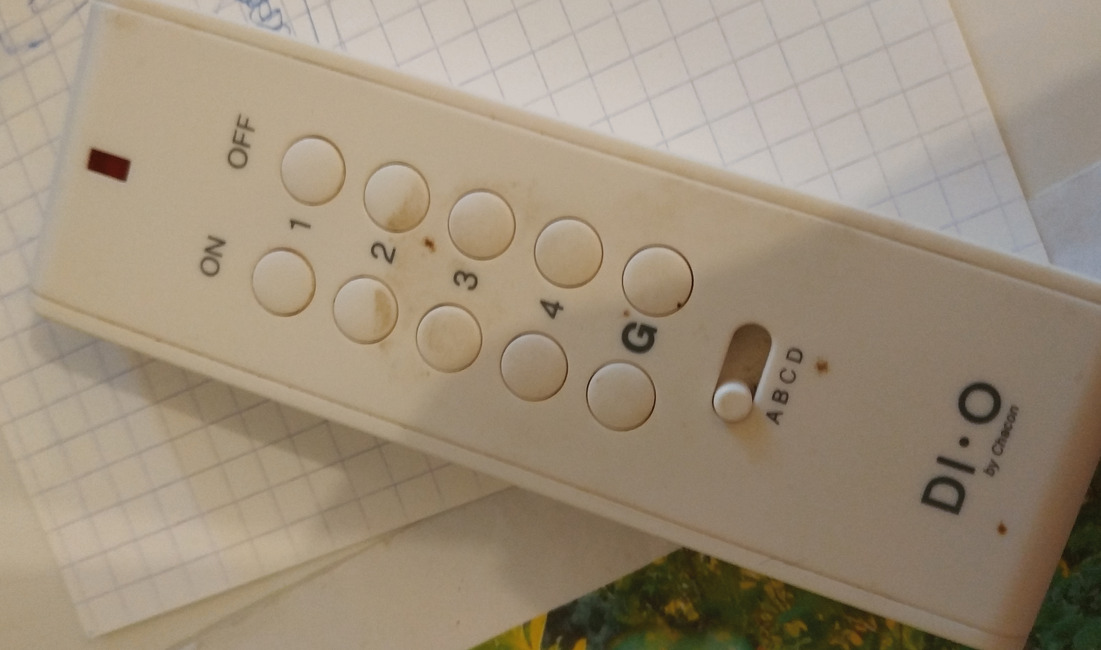
arduino-cli monitor --timestamp --quiet -p "${device}" -b arduino:avr:uno
[2024-12-20 13:29:44] Setting up
I point a remote control to the device and click on a button and… nothing happens.
Hmmm, let’s try to dig into what it does.
The code of rc-switch uses attachInterrupt(), so let’s try to use it.
#include <RCSwitch.h>
RCSwitch mySwitch = RCSwitch();
volatile byte state = LOW;
void handleInterrupt() {
state = !state;
if(state){
Serial.println("1");
} else {
Serial.println("0");
}
}
void setup() {
Serial.begin(9600);
Serial.print("Setting up");
attachInterrupt(digitalPinToInterrupt(2), handleInterrupt, CHANGE);
}
void loop() {
}
Sketch uses 1920 bytes (5%) of program storage space. Maximum is 32256 bytes.
Global variables use 232 bytes (11%) of dynamic memory, leaving 1816 bytes for local variables. Maximum is 2048 bytes.
[92mUsed library[0m [92mVersion[0m [90mPath[0m
[93mrc-switch[0m 2.6.4 [90m/home/sam/Arduino/libraries/rc-switch[0m
[92mUsed platform[0m [92mVersion[0m [90mPath[0m
[93marduino:avr[0m 1.8.6 [90m/home/sam/.arduino15/packages/arduino/hardware/avr/1.8.6[0m
New upload port: /dev/ttyACM1 (serial)
Monitoring the board, I can see a lot of things going on.
:
1[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
[2024-12-20 13:59:07] 0
[2024-12-20 13:59:07] 1
I realized then that this happens:
- no wire connected to the pin
- steady value
- connecting the wire
- going crazy
- shorting the pin with 5V
- steady 1
- shorting the pin with GND
- steady 0
- connecting to the data of the receiver
- going crazy
I don’t understand all those changes, let’s try to see how the other interrupts behave.
#include <RCSwitch.h>
RCSwitch mySwitch = RCSwitch();
volatile byte state = LOW;
void handleInterruptRising() {
Serial.println("Rising");
}
void handleInterruptFalling() {
Serial.println("Falling");
}
void handleInterruptChange() {
Serial.println("Change");
}
void handleInterruptLow() {
Serial.println("Low");
}
void setup() {
Serial.begin(9600);
Serial.print("Setting up");
pinMode(2, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(2), handleInterruptRising, RISING);
attachInterrupt(digitalPinToInterrupt(2), handleInterruptFalling, FALLING);
attachInterrupt(digitalPinToInterrupt(2), handleInterruptLow, LOW);
attachInterrupt(digitalPinToInterrupt(2), handleInterruptChange, CHANGE);
}
void loop() {
}
Sketch uses 2018 bytes (6%) of program storage space. Maximum is 32256 bytes.
Global variables use 236 bytes (11%) of dynamic memory, leaving 1812 bytes for local variables. Maximum is 2048 bytes.
[92mUsed library[0m [92mVersion[0m [90mPath[0m
[93mrc-switch[0m 2.6.4 [90m/home/sam/Arduino/libraries/rc-switch[0m
[92mUsed platform[0m [92mVersion[0m [90mPath[0m
[93marduino:avr[0m 1.8.6 [90m/home/sam/.arduino15/packages/arduino/hardware/avr/1.8.6[0m
New upload port: /dev/ttyACM1 (serial)
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
[2024-12-20 14:07:31] Change
It looks like I can only see the last ICR code, while the documentation says that they would be chained. Switching the “Change” and “Low” lines in the code will result in a lot of “Low” lines in the output.
I appears clearly to me that I don’t understand at all how arduino interruption works.
try reading the values using an analog pin
I plugged the data pin of the receiver on A0 and ran this code.
#include <RCSwitch.h>
RCSwitch mySwitch = RCSwitch();
void setup() {
Serial.begin(9600);
}
void loop() {
float val = analogRead(0);
Serial.println(val);
delay(10); // ms
}
Sketch uses 3504 bytes (10%) of program storage space. Maximum is 32256 bytes.
Global variables use 239 bytes (11%) of dynamic memory, leaving 1809 bytes for local variables. Maximum is 2048 bytes.
[92mUsed library[0m [92mVersion[0m [90mPath[0m
[93mrc-switch[0m 2.6.4 [90m/home/sam/Arduino/libraries/rc-switch[0m
[92mUsed platform[0m [92mVersion[0m [90mPath[0m
[93marduino:avr[0m 1.8.6 [90m/home/sam/.arduino15/packages/arduino/hardware/avr/1.8.6[0m
New upload port: /dev/ttyACM1 (serial)
I ran the program and pressed 3 times the shutter close button, then 3 times the shutter open button and 3 times again the shutter close button.
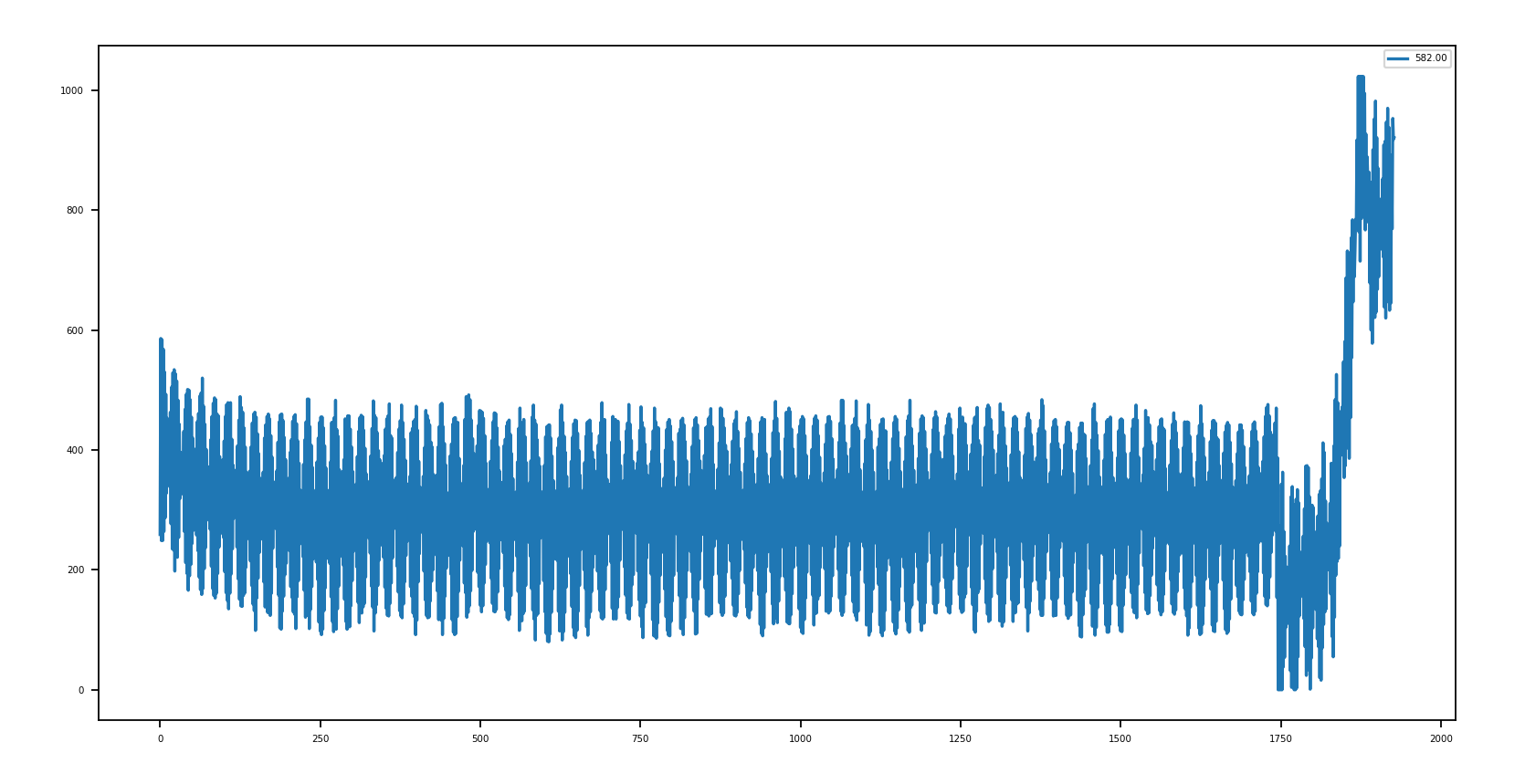
Zooming a bit, I can see this pattern.
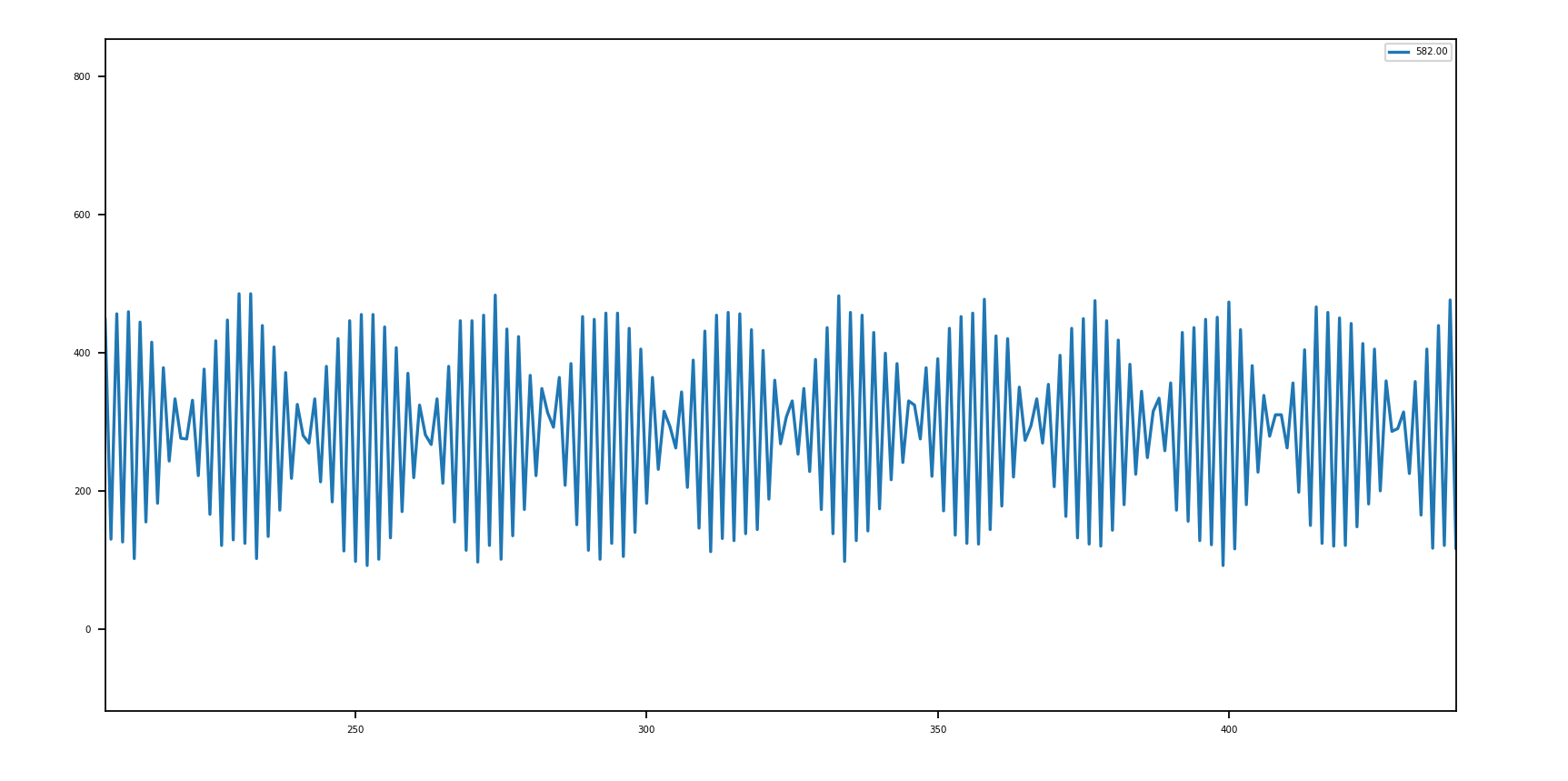
Now, let’s try to disconnect the receiver, keeping the wire dangling.
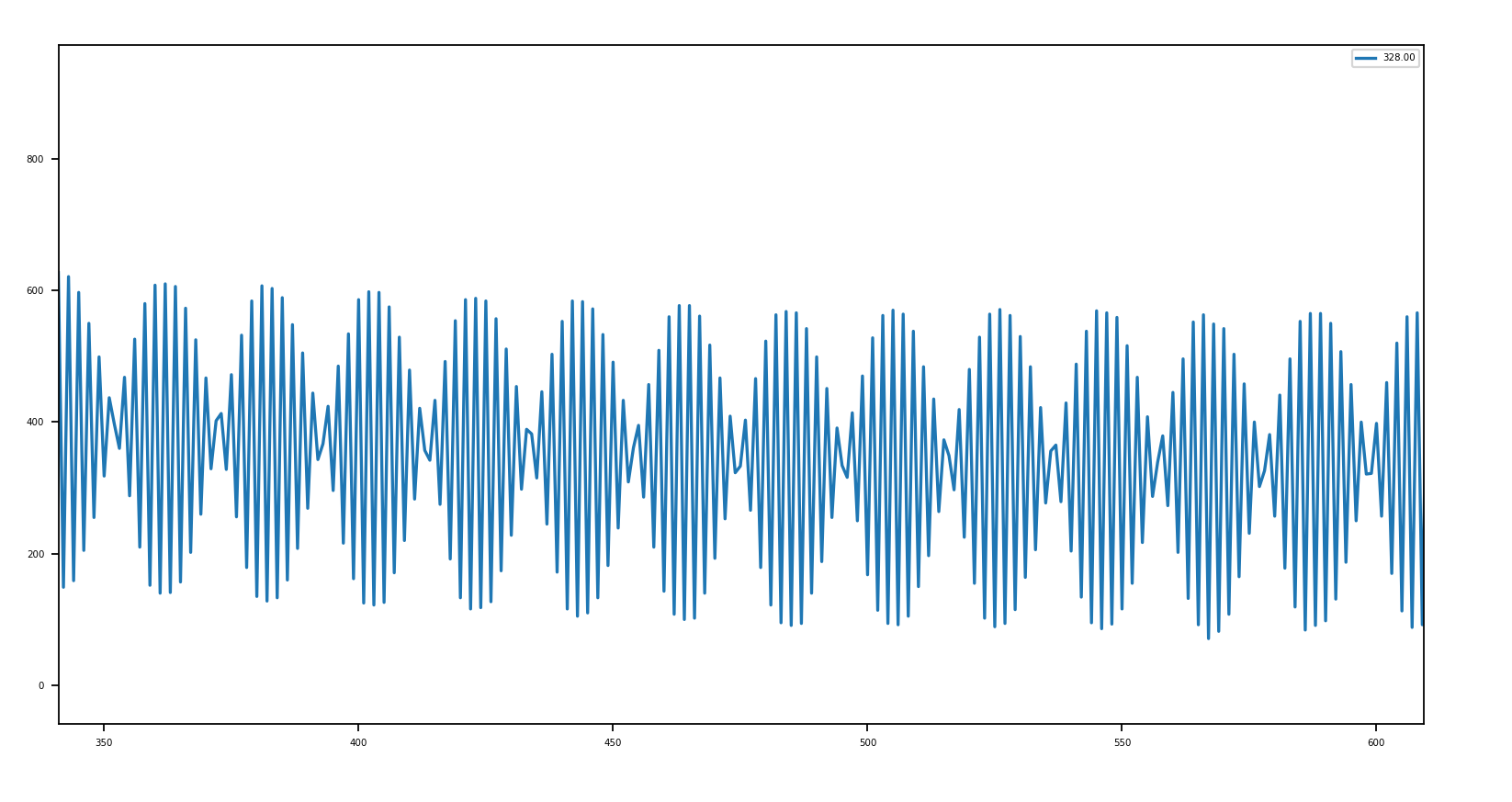
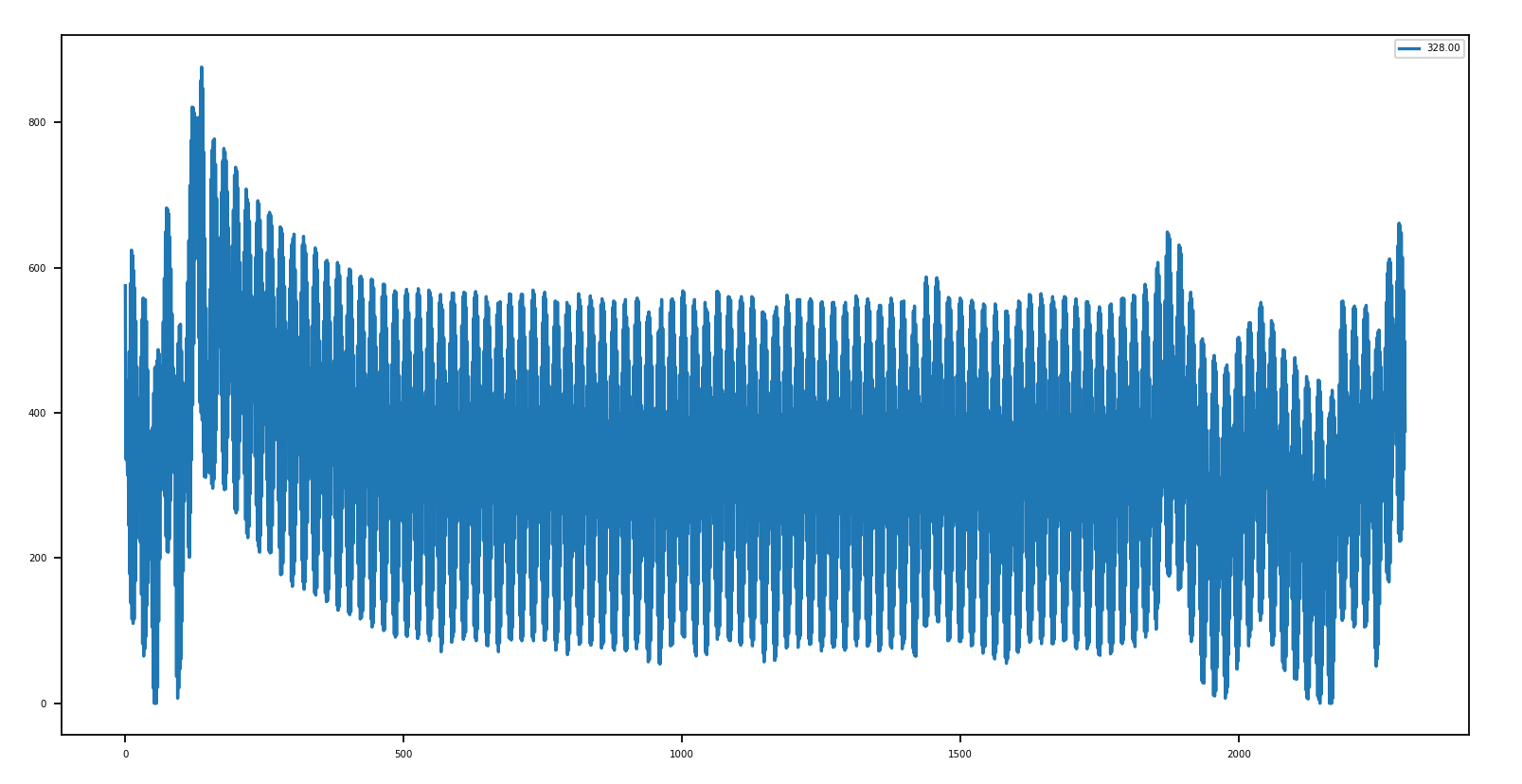
And after removing the wire.
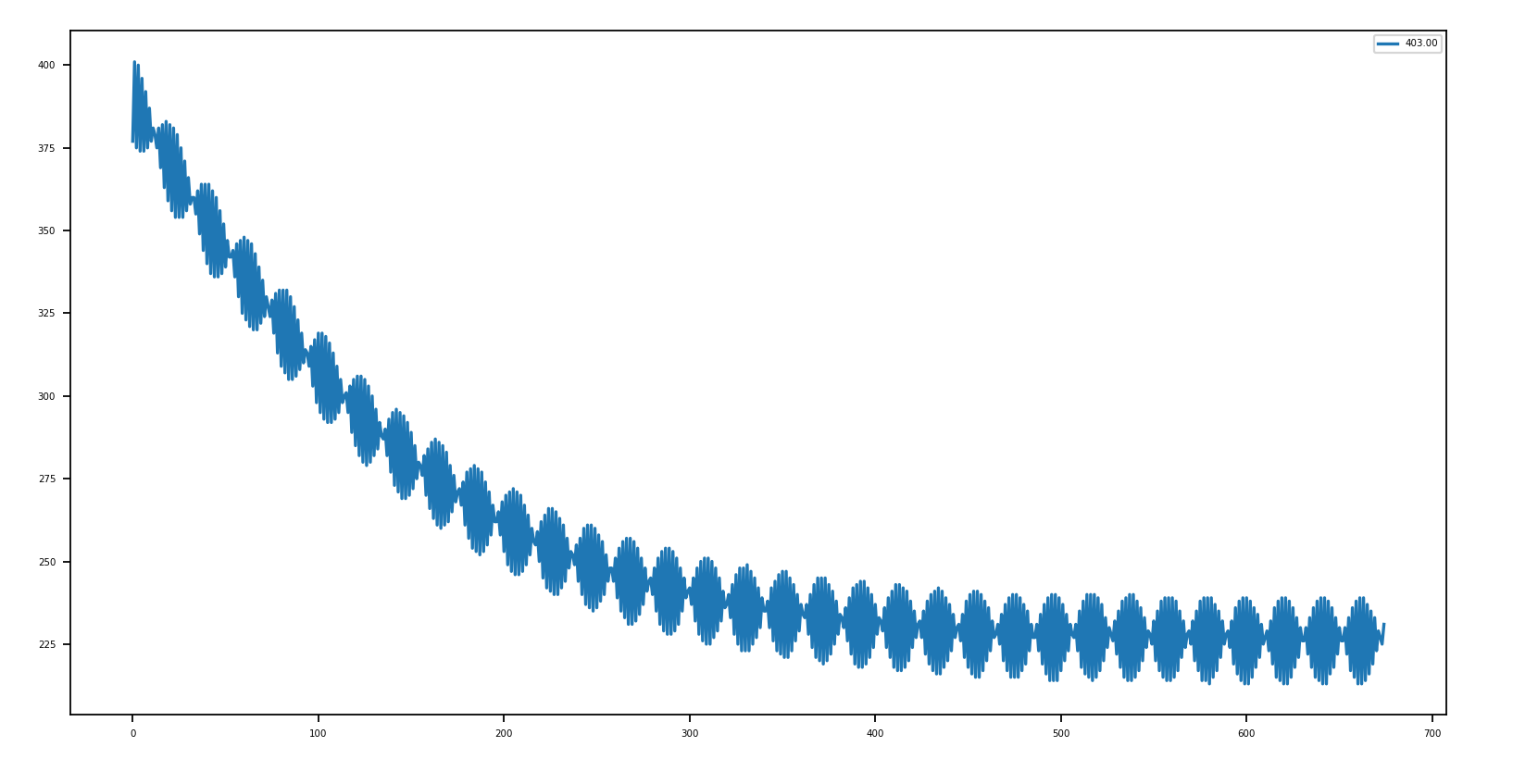
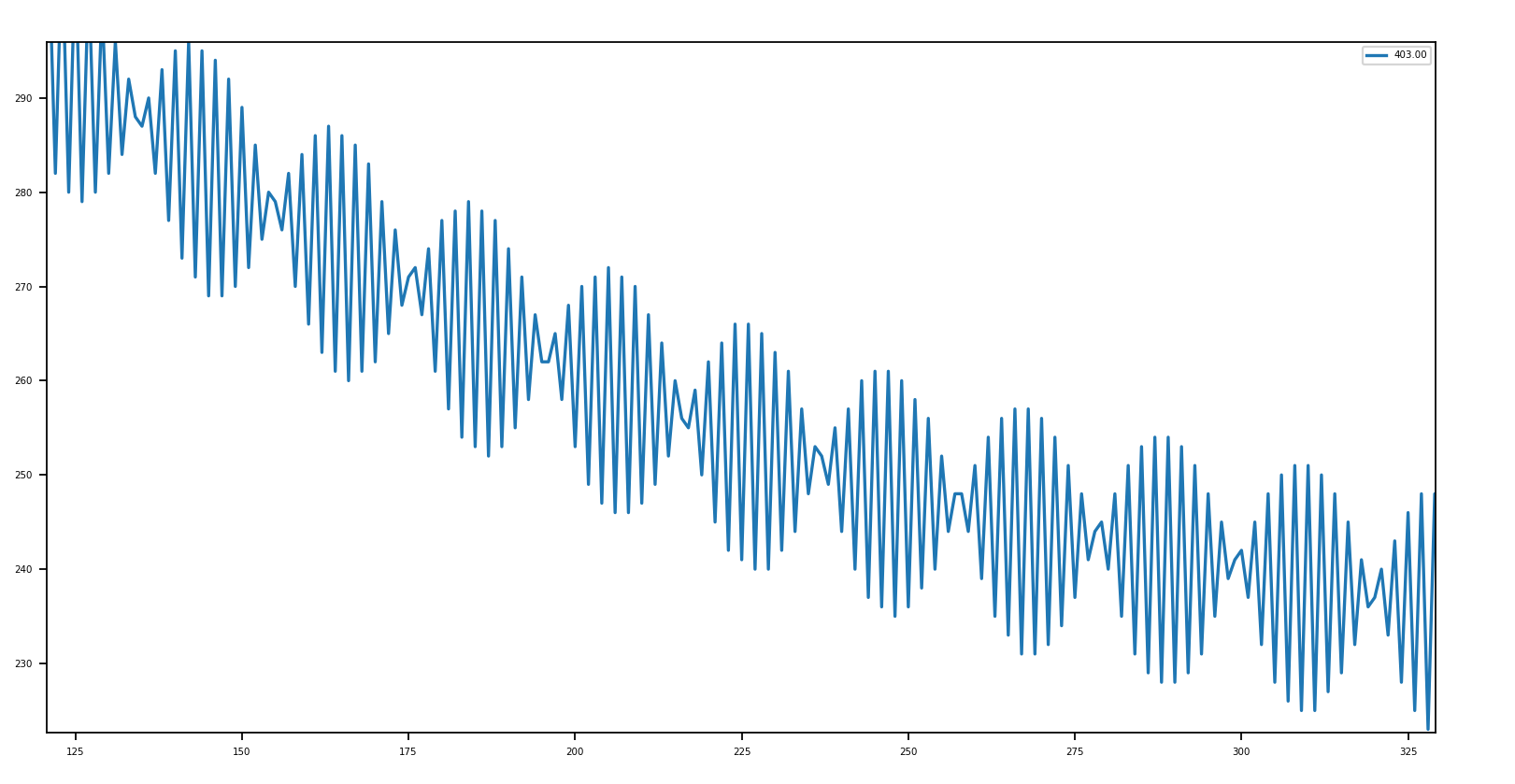
Ok, I definitely got lost. Either my arduino board is broken, or I missed something that will retrospectively appear obvious.